Earlier we saw how we can map our entities and Database using code first approach .
Here I have created a simple demo to show how already existing database table can be mapped to our model class entities
To do that , I created a table in MS SQL server 2012 called "Products"
Now , in visual studio project. Add new item(Ado.net entity data model) under model folder
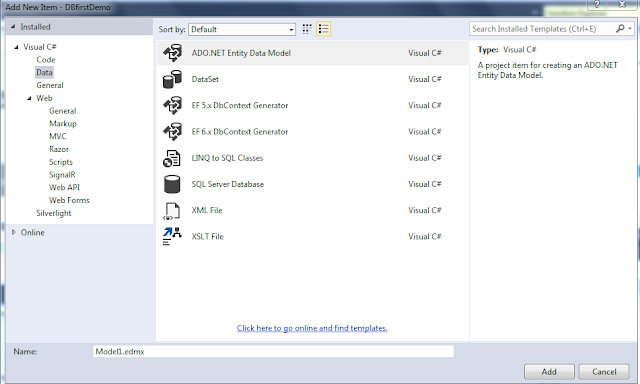
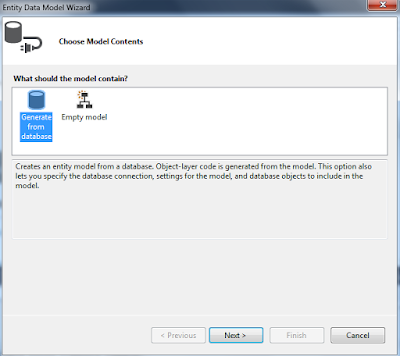
Create a new SQL connection
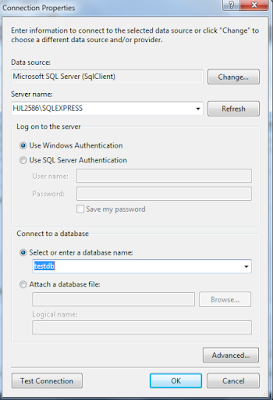
Select the db objects like tables,views,sp.. whichever is relevant
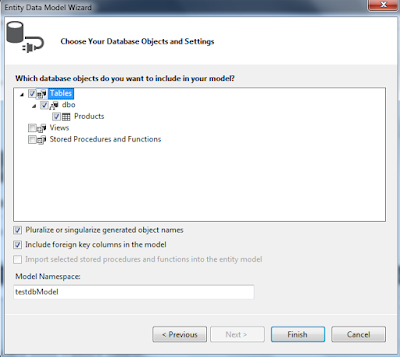
Your designer class will be ready to show the entity diagram
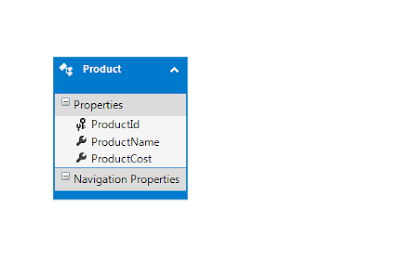
Now you can see the database table schema is mapped to model classes. POCO classes are created automatically by entity framework along with context class
Now add a controller and choose scaffolding template .
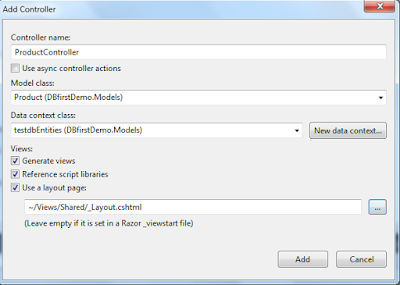
Controller action methods are as below
Views are automatically created . build the solution and run it.
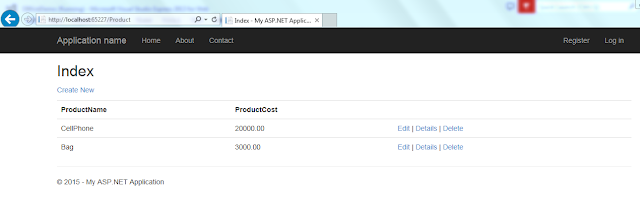
you can do CRUD operations .
I have added an item using create new link on the UI
and same is reflected in the DB
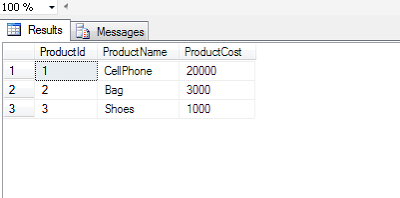
Here I have created a simple demo to show how already existing database table can be mapped to our model class entities
To do that , I created a table in MS SQL server 2012 called "Products"
Now , in visual studio project. Add new item(Ado.net entity data model) under model folder
Create a new SQL connection
Select the db objects like tables,views,sp.. whichever is relevant
Your designer class will be ready to show the entity diagram
Now you can see the database table schema is mapped to model classes. POCO classes are created automatically by entity framework along with context class
public partial class Product
{
public string ProductId { get; set; }
public string ProductName { get; set; }
public Nullable<decimal> ProductCost { get; set; }
}
Now add a controller and choose scaffolding template .
Controller action methods are as below
public class ProductController : Controller
{
private testdbEntities db = new testdbEntities();
// GET:
/Product/
public ActionResult Index()
{
return View(db.Products.ToList());
}
// GET:
/Product/Details/5
public ActionResult Details(string id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Product product = db.Products.Find(id);
if (product == null)
{
return HttpNotFound();
}
return View(product);
}
// GET:
/Product/Create
public ActionResult Create()
{
return View();
}
// POST:
/Product/Create
// To
protect from overposting attacks, please enable the specific properties you
want to bind to, for
// more
details see http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create([Bind(Include="ProductId,ProductName,ProductCost")] Product product)
{
if (ModelState.IsValid)
{
db.Products.Add(product);
db.SaveChanges();
return RedirectToAction("Index");
}
return View(product);
}
// GET:
/Product/Edit/5
public ActionResult Edit(string id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Product product = db.Products.Find(id);
if (product == null)
{
return HttpNotFound();
}
return View(product);
}
// POST:
/Product/Edit/5
// To
protect from overposting attacks, please enable the specific properties you
want to bind to, for
// more
details see http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit([Bind(Include="ProductId,ProductName,ProductCost")] Product product)
{
if (ModelState.IsValid)
{
db.Entry(product).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
return View(product);
}
// GET:
/Product/Delete/5
public ActionResult Delete(string id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Product product = db.Products.Find(id);
if (product == null)
{
return HttpNotFound();
}
return View(product);
}
// POST:
/Product/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult DeleteConfirmed(string id)
{
Product product = db.Products.Find(id);
db.Products.Remove(product);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
}
Views are automatically created . build the solution and run it.
you can do CRUD operations .
I have added an item using create new link on the UI
and same is reflected in the DB